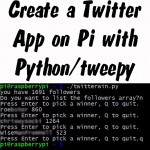
I wanted to be able to interact with my twitter account(s) using Python scripts. I’d heard there was a Python library called tweepy that does a lot of the Applications Programming Interface (API) work for you. I’d also seen quite a few posts in the Python section of the Raspberry Pi Forums, where people had problems with it.
Prize Draw
I thought I’d take a look, spurred on by the idea that I’d like to be able to select a random twitter follower every once-in-a-while for a prize draw.
So, last week, I spent a morning on this. The biggest problem I had was that, following the instructions for basic authentication “it just didn’t work”. I knew that twitter had changed its API over the summer because the twitter widget on the RasPi.TV blog stopped working. I had to go and created my own widget on twitter to make it work again. Could it be related to that?
You can’t use tweepy without OAuth authentication
It certainly could and it certainly was. In fact, the problem was that the tweepy documentation was hopelessly out of date. This basic authentication is irrevocably broken and will never work again.
But you know how it is. People who like programming, often don’t like documenting. And that leaves people like you and I up the proverbial creek without a paddle. We can either give up, or try and see if other people are having the same issues and how they solved it. A second round of googling was required.
I eventually found a blog that got me through the process (although sadly I forgot to bookmark it and can no longer find it). In fact, it’s rather easy – when you know how! And I’m going to share it here with you, so you don’t have to suffer the same wasted couple of hours I had to.
Create an ‘App’
The first thing you have to do is create an app for the twitter API. Sounds scary, but it’s not. Just go to https://apps.twitter.com/ and log in with your twitter account id and password.
Once logged in, hover over your avatar and click My Applications, then click Create a new Application
Then you’ll have to complete four fields in the form. Name, Description, website and callback URL. The last two don’t much matter for what we’re doing here.
Then you have to tick the box agreeing to their terms and then complete the CAPTCHA. Then click Create Your Twitter Application.
Find Your App
Hover over your avatar and click on “My Applications”.
You should now see a page with all your created twitter apps. Click on your new app.
You should then see something like this…
It creates a “Read only” app by default. You can change that later if you want (by clicking the OAuth tab) but we’ll leave it as is for now because we only need read-only access for this program.
Further down the page it looks like this…
Create Access Token
In order for your program to talk to twitter, it needs a way of authenticating. The system used is called OAuth, and it uses access tokens (codes). So you need to click the “Create My Access token” button…
…to make this happen. Soon after that, you should have an additional section at the bottom of the page (you might need to refresh the page (F5) to see it)…
Keep this page open. You will need to copy these codes into your program.
Install tweepy
Before you can use tweepy, you need to get it onto your Pi. Fortunately, it’s a Python extension, so easy to install.
sudo apt-get update
updates Raspbian package lists
sudo apt-get install python-dev python-pip
install pip and dev (You may not need python-dev for pip and tweepy, but I installed it anyway.)
Update Jan 2015: I’ve just tried to install tweepy and it failed. The reason appears to be an older version of pip in the Raspbian repo. You can get round this with…
sudo pip install -U pip
(this will uninstall pip and replace it with a version that works).
…before you install tweepy.
sudo pip install tweepy
install tweepy itself
You now have all you need to make your Python script talk to twitter.
All you need now is a Python script
Here’s the script I wrote to choose a random subscriber from my list of twitter followers.
To make this script work on your system, you’ll need to edit lines 7-10 to include your keys, secrets and tokens.
#!/usr/bin/env python2.7 # twitterwin.py by Alex Eames https://raspi.tv/?p=5281 import tweepy import random # Consumer keys and access tokens, used for OAuth consumer_key = 'copy your consumer key here' consumer_secret = 'copy your consumer secret here' access_token = 'copy your access token here' access_token_secret = 'copy your access token secret here' # OAuth process, using the keys and tokens auth = tweepy.OAuthHandler(consumer_key, consumer_secret) auth.set_access_token(access_token, access_token_secret) # Creation of the actual interface, using authentication api = tweepy.API(auth) follow2 = api.followers_ids() # gives a list of followers ids print "you have %d followers" % len(follow2) show_list = raw_input("Do you want to list the followers array?") if show_list in ('y', 'yes', 'Y', 'Yes', 'YES'): print follow2 def pick_winner(): random_number = random.randint(0, len(follow2)-1) winner = api.get_user(follow2[random_number]) print winner.screen_name, random_number while True: pick = raw_input("Press Enter to pick a winner, Q to quit.") if pick in ('q', 'Q','quit', 'QUIT', 'Quit'): break pick_winner()
I called this program twitterwin.py because I wrote it to pick a winner from my twitter subscribers list.
Update Jan 2016
This code works well up to 5000 twitter followers. For >5000 followers you need to tweak the code a bit. Full details and code in this blog article here. Also tweaked a couple of lines in the code to eliminate some “bad habits” or “unnecessarily clumsy” code.
To run the program, just type
python twitterwin.py
This is what the output looks like. I chose “No” when it asked if I want to see the list of subscribers, as it’s not very pretty or useful, except for debugging. :)
Apologies to the abovementioned twitter members. This was just a test, not a real draw.
Where next?
In part 2, I’ll show you how to use your Python program “app” to send a tweet.
What about the Twitter Draw?
I’ll be announcing this in due course, but for now, make sure you follow @RasPiTV to be in with a chance of winning something. :) That’s all I’m saying about that for now.
I connected twitter up to a stepper motor to spell out tweets, using methods like this
http://instagram.com/p/dXcNibGj5G/
That’s clever :)
I had a idea to post “quote of the day” from the application Fortune. But i’m a newbie in python-tweepy and perhaps someone can help me get started?
Part 2 will show you how to make your app read/write and post tweets with it. That should get you part of the way there, but make sure anything you’re posting isn’t copyright or you could get in hot water :)
Getting the following error:
File “/home/pi/work/python/tweepy.py”, line 13, in
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
AttributeError: ‘module’ object has no attribute ‘OAuthHandler’
Leo
Sorted. – I was being an idiot! Should not have named my program the same name as the module I was importing. :-)
Leo
we’ve all done it :)
I had a problem once when I named a script email.py. How the heck was I supposed to know there was a module of that name? Changed the script name and it worked.
Thanks for this, I’ve got my RPi using MariaDB on my Ubuntu server with peewee to get the consumer key and consumer secret and tweeting with tweepy.
Sending the tweet was trivial, but I’ll wait for part II.
Sorry, I’ve been distracted by Pi NOIR and our KickStarter. I agree, actually sending the tweet is not hard.
very nice & helpful blog. If I need to get the tweets as it is from my account what extra lib should I use?
Thank you
Hi. This is actually quite simple and I plan a blog about it, but at the moment, my focus has had to be on the time limited KickStarter campaign for HDMIPi.
http://pythonhosted.org/tweepy/html/api.html#status-methods shows the commands though.
api.get_status()
is the one you wanthi there. you can make twitter app with midori.
it’s very useful/fast app…
http://www.raspberrypi.org/forum/viewtopic.php?f=89&t=63801
Perhaps I’ve missed the point, but it doesn’t look like that’s quite what I was doing.
Hi.
Im trying to post a tweet, but i have some troubles:
api.update_status(tweet_text)
So, i can not put any tweet.
[…] un nuovo account Twitter e, seguendo questa breve guida, otteniamo tutte le chiavi di autorizzazione per poter utilizzare il nostro nuovo account Twitter […]
[…] Installed Tweepy (and pip) for sending tweets from python […]
[…] came across a nice tutorial about how to create a twitter app, and followed most part of it. In the below, we will detail what […]
[…] is done wrong you may break other alerts that are already setup. I really need to thank https://raspi.tv/2013/how-to-create-a-twitter-app-on-the-raspberry-pi-with-python-tweepy-part-1#insta… here as I used this as a starting […]