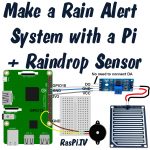
You’ve been left at home in charge of the laundry. It’s already washed and hanging out to dry on the line. But you were warned – on pain of death – to bring it in if it starts to rain. The trouble is, you’re totally into whatever it is you’re coding right now and you won’t even notice if it rains. You’ll be toast if it rains and the laundry gets wet. So which is it to be? Code or laundry? That was your choice – until now!
Let’s Make a Rain Alert System
We’re going to use a simple raindrop sensor, a buzzer and a Pi to alert you when it rains. So you can get on with something useful instead of watching the weather. The components can all be found in a little kit on the RasPiO online shop.
You could also use an Arduino or Wemos, but a Pi will let us use GPIO Zero with Python and will be easier to extend later if we want to take it further (e.g. sending tweets etc.).
To get an alert when it starts to rain, we’re going to rig this sensor up to a Raspberry Pi and trigger a buzzer. You could also have it blink LEDs, send you an SMS or tweet a photo of the washing line and the sky. But we’re going with a buzzer for now.
Here’s the Circuit
And this is what the real thing looks like when it’s all hooked up to the Pi. I’m using a Pi3B but you could use any model of Pi for this.
Here’s the Python Code
# raindrop sensor DO connected to GPIO18 # HIGH = no rain, LOW = rain detected # Buzzer on GPIO13 from time import sleep from gpiozero import Buzzer, InputDevice buzz = Buzzer(13) no_rain = InputDevice(18) def buzz_now(iterations): for x in range(iterations): buzz.on() sleep(0.1) buzz.off() sleep(0.1) while True: if not no_rain.is_active: print("It's raining - get the washing in!") buzz_now(5) # insert your other code or functions here # e.g. tweet, SMS, email, take a photo etc. sleep(1)
When no raindrops are on the sensor, the sensor controller’s DO (digital out) pin is HIGH (3.3V in our case). When raindrops are detected this changes to LOW (0V). By connecting DO to a GPIO port on the Pi (GPIO18) we can read the status and set off the buzzer (on GPIO13) when rain is detected.
When rain is detected, the buzzer is sounded 5 times in quick succession, followed by a 1s pause. This will repeat until the raindrops are no longer detected. A message will also be printed out on the screen each second while raindrops are detected…
Calibration
You want the sensor to trigger when you have a couple of drops of water on it. It’s up to you exactly how many. I prefer it when it’s 2-3 drops or one really large one. You can change the trigger sensitivity by twiddling the controller board potentiometer when you have drops on your sensor. With your chosen number of drops on the sensor (and the Python script running) twiddle the pot. until the buzzer stops beeping and then back off until it just starts again. Then you have your setting. Then tweak and play with it as much as you wish to makes sure it works as you want.
Then put the Pi and controller in some kind of box to protect it from the rain, but obviously leave the sensor itself exposed. Run the python script and wait for the beeps to tell you it’s raining.
Suggestions for Taking it Further
- add some LEDs and make them activate when it rains
- send an email warning when it starts to rain
- send an SMS when it starts to rain
- send a push notification to your phone when it rains
- tweet at you when it starts to rain
- tweet a photo of the washing line and the sky when it starts to rain
- tweak the code so that it won’t keep beeping for the whole time it rains (that will grow old quickly)
If You’d Like to Make One
I’ve put together a little kit containing the parts you need for this project. If you’d like one, you can buy them from the RasPiO online shop here.
Kit Contents
- Raindrop sensing board
- Control board
- 5 jumper wires with F-F DuPont connectors
- 10 jumper wires with M-F DuPont connectors
- Buzzer (active piezo)
- Mini breadboard
I guess an easy extension to this project would be to add a push-button to signify “I’ve now got the washing in” :-)
I haven’t tested this, but I believe your buzz_now(5) function could be replaced by buzz.beep(0.1, 0.1, 5)
And it’s only a small difference, but if you used DigitalInputDevice instead of InputDevice then you’d be able to use https://gpiozero.readthedocs.io/en/stable/api_generic.html#gpiozero.EventsMixin.wait_for_inactive instead of having to sleep(1) inside a while True: loop.
Good suggestions :) Thanks Andrew. You were up late this morning.
An interesting basic project – but has a large drawback. After each shower one must run outside and remove any water left on the sensor board. Perhaps by reading raindrops falling through a tube? This would be excellent for indoor work like monitoring a washing to sound an alarm if it loses water.
Yes I agree it has its limitations, but it’s rather fun and you could get it to ‘ignore’ signals for a time in software after the initial alert. It’s ideal for a one shot warning, e.g. the ‘washing line’ scenario above, or if you’ve painted something and left it drying outside and want a warning to go and cover it or bring it inside to protect from rain.
Coupled with a Pi camera, you’d be able to get a visual even if you weren’t on site. That’s why I chose a Pi not an Arduino. :)
Or you could just use attach a servo and have that “wipe” the rain sensor clean ;-D
What’s scary is that I almost said that too. Or a servo to tilt the sensor to tip the water off so it clears faster.
Previously i can run this program then after i run upgrade python it shows below error. Can you please help me.
Traceback (most recent call last):
File “/home/pi/rainsensor.py”, line 5, in
from gpiozero import Buzzer, InputDevice
File “/usr/lib/python3/dist-packages/gpiozero/__init__.py”, line 22, in
from .devices import (
File “/usr/lib/python3/dist-packages/gpiozero/devices.py”, line 19, in
import pkg_resources
File “/usr/lib/python3/dist-packages/pkg_resources/__init__.py”, line 36, in
import email.parser
ImportError: bad magic number in ’email’: b’\x03\xf3\r\n
No idea – sorry – looks like the upgrade has messed something up on your system. I’d suggest using a fresh install of the latest Raspbian which will have the latest python and GPIO Zero libraries which work together properly.
I built something like that years ago which I used to automatically close some skylights. The issue with it was that the sensor started corroding after a few months of exposure to the elements and become erratic. I ended up with false alarms and worse, no alarm at all.
couple of thoughts on rain clearing – put the whole mechanism on a rotating table and spin the water off… or a heated blower triggered after a delay, or a heated pad under the sensor to evaporate the water triggered after a delay. As a one-shot notification though, what a great idea :-).
Yes I think of it more as a one-shot notification device. I don’t know how long the sensor would last if permanently exposed.
Nice post, but keeping RPi running only for this purpose is an overkill. Esp8266 alone will be more than enough.
Horses for courses – there’s probably many more RPi owners than there are Esp8266 owners :-)
You could even argue that “keeping Esp8266 running only for this purpose is an overkill. Looking out of the window alone will be more than enough.” ;-D
Or just stay outside and feel the first drop, then “don’t panic Mr Mainwaring!”
I have a Velux rain sensor that uses 24V because it has a heating plate. Is there any way to get this one running (no binary out, but analog signal)?
There is three wires on it, so it seems (I haven’t disassembled it as it’s likely to destroy the water-proofness) that the ground line is shared. Is there any way to get this running (if not with a Pi with any board that would work on 24V?)?
great little kit ,bought it months ag ,i finally got around to try it ,and a few tweaks on the blue dial and it worked great ,great price too
I need a rain sensor that I can rely on all season, day and night. It needs to be installed somewhere around my house.
How does this sensor compare to the kind which are assembled on top of a water tight electricity box, and which have a small heating device in them to dry the sensor after the rain has finished?
Without any water drop it started buzzing . And how to stop the func ?