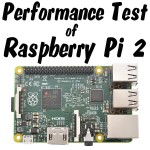
The new Raspberry Pi 2 has a quad-core CPU (BCM2836), but it costs the same as the previous B+. All that extra CPU power isn’t a completely free lunch though. If you use it, it’ll cost you slightly more electrical power than the B+. But how much? That’s what we’re here to look at. In my testing, hammering different numbers of cores in parallel, it worked out at roughly 50 mA (250 mW) per core.
But before I show you the full results, let me back up and show you how we got them because the method is as interesting as the results.
Today we’re testing how much faster the quad-core A7 BCM2836 processor is than the single-core BCM2835. I’ve also done some performance measurements on an Allwinner A20-based (dual A7) processor for fun. But while testing these performance gains, I’ve measured the power usage as well.
How Did I Hammer The CPUs?
Back in early 2012, inspired by Pi, I started learning Python while waiting for the Pi to arrive. Everyone said “choose a project – it’s the best way to learn”. So after I’d learnt the basics, I decided to write a program to help me beat my friends at “Words With Friends”. The idea was you punch in the letters you have and it would search through the entire word list and give you a list of possible words you could use.
I got it working, but it was horribly slow and inefficient, even on a PC. It took a couple of minutes to run. I eventually came across the idea of pre-sorting the list of 172,820 words so that the computer could scan it more efficiently. This pre-processing only needed to happen once and it sped up the actual search to just a handful of seconds. It even ran acceptably fast on the Pi, which is why I’ve chosen this pre-sorting program to test the difference in CPUs. 20-30 seconds is a nice easy time period to measure.
But that left just one issue. To test the quad-core processor properly we need to be able to run this process 1, 2, 3, & 4 times at once, preferably at will. To do this, we need to be able to run multiple threads simultaneously. I’d used threading once before, but found it a bit fiddly. The online documentation sucked (why so sparse with the examples – documenters?), but I eventually found an example here that I was able to adapt for my own needs. I love examples. Please include lots of examples if you ever document anything. :) Here’s the code I used to drive the threading…
The Code
#!/usr/bin/env python2.7 # script by Alex Eames https://raspi.tv/?p=7684 import time from subprocess import call from threading import Thread cmd = "python /home/pi/presort.py" def process_thread(i): print "Thread: %d" % i start_time = time.time() call ([cmd], shell=True) end_time = time.time() elapsed_time = end_time - start_time print "Thread %s took %.2f seconds" % (i, elapsed_time) how_many = int(raw_input("How many threads?\n>")) for i in range(how_many): t = Thread(target=process_thread, args=(i,)) t.start()
All it does is ask you how many threads you want (how_many) and then call the presorting script how_many times, running each one in a different thread. It isn’t bullet-proof (there is no error-checking on the input), but I don’t care! It does what it’s meant to.
From what I can gather from the results and the current measurements, each thread is running on a different CPU when we use multi-core processors. So this is a great way to hammer as many or as few CPUs at a time as you choose. :)
What About The Results?
Performance Results
The B+ can run this pre-sorting script once in 26 seconds or twice simultaneously in about 53 seconds.
The new Pi2 can run it once in about 7.4 seconds, but because it’s quad-core it can actually run it 4 times in parallel in 7.7 seconds as well.
So not only is the ARMv7 BCM2836 3.5 times faster than the BCM2835’s old ARMv6, but there’s four of them so, for this example it can work 3.5 x 4 = 14 times faster than the B+
While I was at it I ran the same program on an A20 based board (nana). For one thread it took 7.5s. Two threads took 9.5 seconds. Four threads was roughly double that time (it’s dual-core).
As a point of reference, my MacBook Pro ran a single thread in 0.63s (gotta love the cross-platform nature of Python).
Power Results
The measured current when using the presort.py script to hammer varying CPUs on the Pi2 at 5.18-5.19V
So you can see that each CPU uses ~40-50 mA when being pushed.
I Also Did My Standard Pi Test Series
The ‘standard’ set of measurements that I do on new Pis does not incorporate multi-core, but I did them anyway, so we can have an accurate comparison of all Pis. The lack of a multi-core test is why I created one above.
Looking at these data, the Pi 2 appears to use a little more than a B+. But since these data are all single-core tests, if we added in another 150 mA to simulate the hammering of all four cores, the numbers would be very similar to those of the model B. I will have to devise a test which shoots 1080p video while hammering all four cores. But that’s for another occasion.
If You Want To Try It
I’ve shared the code for this on GitHub if you want to have a go. To run this on your Pi…
git clone https://github.com/raspitv/pi2test/
cd pi2test
python Pi2test.py
Have fun.
Results of i5-4570 CPU:
$ python Pi2test.py
How many threads?
>1
Thread: 0
Starting now…
Finished. That took 0.44 seconds
Thread 0 took 0.51 seconds
$ python Pi2test.py
How many threads?
>4
Thread: 0
Thread: 1
Thread: 2
Thread: 3
Starting now…
Starting now…
Starting now…
Starting now…
Finished. That took 0.58 seconds
Finished. That took 0.58 seconds
Finished. That took 0.58 seconds
Finished. That took 0.61 seconds
Thread 2 took 0.75 seconds
Thread 0 took 0.76 seconds
Thread 1 took 0.78 seconds
Thread 3 took 0.77 seconds
Similar to my MacBook :) I think with faster processors the IO might be the limiting factor? WJDK.
I might try running this from a RAMDisk when I get my Pi2. It’s not like it’ll be short of memory… ;-)
nice one!
on AMD E-450 I got 2.12 secs for 1thread, 2.16 for 2threads – looks like if compared vs older dual core atoms, they should be 3-4x of pi2 on average (and of course let’s not mention power consumption :) )
still if somebody wants to try this on atom netbook etc, then I would be very happy to see the score posted here :)
[…] Eames from RasPi.TV has written a Python script that tests out the capabilities of the Raspberry Pi 2’s quad-core […]
That’s brilliant, thank-you. Would you be able to re-run the tests with a common Ralink 5370 USB WiFi adapter running please? My understanding is that WiFi can draw quite an additional current, and it is a very common device to have attached. Whereas a USB keyboard and mouse consumes next to nothing. Of course I suppose it depends upon the network load, though.
I’ve never managed to make any wifi dongle draw more than about 100 mA. Normal use something in the region of about 50-60.
“All that extra CPU power isn’t a completely free lunch though… it worked out at roughly 50 mA (250 mW) per core.”
LOL, I don’t think I’ll be worrying too much then about the impact of the Pi2 on my electricity bill ;-)
No it’s still a very cheap lunch
The classic test is calculating prime numbers. Just a suggestion, but how about reading the file in its entirety – passing it as an object to the threads before you start them off – then you can take I/O out of the output figures.
Hmmm, looking at presort.py on your github I see that each of the 4 threads will be trying to write to the same filenames, which might be why using 4 threads on the Pi2 is slightly slower than 1 thread – each thread will block trying to open the file for writing, until whichever thread has the file currently open-for-writing has closed it.
And the code would probably also be a bit faster if you simply saved the results from the first ‘sort pass’ into a python list as you go, rather than writing the results out to /home/pi/sortedwordlist.txt and then reading the file back in again just a few lines later ;)
And finally it’s considered “more pythonic” to do
(the latter version will obviously use more memory, but big writes are more efficient than lots of small writes).
These are all good things to learn. Good points.
Remember though, the original script was written to do a one-off sort, three years ago when I was learning. Pretty sure I mentioned that in the article ;p
Its definitely not an example of “the best way to code”.
Could re-take the measurements with updated code to stress the filesystem/memory card less?
Yep I did think about what difference taking the I/O out of it might make – thanks :)
Based on your python code, I doubt you actually using all processor cores because Python threading module can’t handle multiprocessor. It’s Python’s limitation due to Global Interpreter Lock. Use multiprocessing module instead. See: https://docs.python.org/3/library/multiprocessing.html#module-multiprocessing
You might be right. I don’t really know. But how do you explain the increased power consumption between running 1,2,3 and 4 threads if it’s just using one core?
The threading script is calling four separate python processes. Surely those can be run on separate cores?
I forgot you used subprocess.call function. With that, you used one or more processors (not by your script, but by operating system).
Yeah, if you took out the time-measuring code I suspect Alex’s Pi2Test.py would be equivalent to this shell script:
#!/bin/bash
python /home/pi/presort.py&
python /home/pi/presort.py&
python /home/pi/presort.py&
python /home/pi/presort.py
Alex, thanks for a nice and simple script.
Mukti, I am little confused. do you mean the script is using multicore ?
Don’t be confused by Mukti’s comment. He overlooked the fact that four separate calls to a Python script were being made. As Andrew says above, the multicore processing side of it is being handled by Linux, which pushes each call out to a different processor.
Thanks Alex to know that is really using all 4 cores.
Side question. Is there a way to know which call ran on which core ? Can we get a printout programatically?
This is purely a guess, but accessing that kind of low-level info might require the use of C rather than Python? (I don’t have much experience with multi-threaded / multi-core Python code)
Good piece. Now another question, how mature do you feel the power management code is for the Pi2? It seems pretty good but I would think there should be room for improvement? Is it likely that the underlying OS still needs to mature a bit in how it handles low use states?
Sorry. I really don’t know. I suggest asking that one on the Raspberry Pi Foundation forums.
I don’t know either, but with the idle power of the Pi2 being so close to the idle power of the B+, I dunno how much more could be ‘saved’ anyway?
I believe there’s already a load of power-saving done in the GPU side, and that’s obviously unchanged between BCM2835 on B+ and BCM2836 on Pi2.
And I guess the “taller peak” for loading LXDE on the Pi2 vs. B+ is also a “narrower peak” because LXDE loads much quicker? ;)
Yes it is. Blink and you miss it :)
Thanks for this great test.
Off topic, I know, but have you tried any GPIO?
An identical low level ‘C’ program that toggles GPIOs 16 through 20 works on B+ produces nothing on Pi2 that I can detect, or am I missing something?
Derek
If using sysfs it should “just work”, but if using memory-mapped access you’ll need to adjust the base address, as http://jamesrandominfo.blogspot.co.uk/2015/02/raspberry-pi-2-colated-faqs.html mentions. http://www.raspberrypi.org/raspberry-pi-2-on-sale/#comment-1198868 has more info.
Thanks Andrew, that was it. I was using mapped memory, I found the call to get the base address but so far have not found which library its in so the repaired code won’t link. If I manually set the address it works as expected, but of course then it wouldn’t be backwards compatible.
I’ll take a look to see which is more efficient between sysfs and memory mapped IO before closing a final fix.
Thanks again for the insight.
If you look at the userland commit that I indirectly linked to, the “-lbcm_host” in host_applications/linux/apps/hello_pi/hello_fft/makefile seems to be what you’re looking for? Additionally you need to ensure that (on both Pi1 and Pi2) DeviceTree is enabled and working (by looking if /proc/device-tree exists). More docs at http://www.raspberrypi.org/documentation/configuration/device-tree.md
Memory-mapped IO is definitely more efficient than sysfs, but using sysfs is more portable (and easier to use) – it’s one of those classic trade-offs ;-)
RPi.GPIO (the Python module that Alex has written many tutorials about) was originally using sysfs, but then moved to memory-mapped, because it’s faster, and also offers access to additional functionality (e.g. interrupts). Additionally, using memory-mapped IO requires your program to always be run as root, whereas sysfs just uses the standard linux file permissions.
I guess you’ll want to keep an eye on https://github.com/raspberrypi/documentation/issues/158
:-)
I made one little change to Hussam Al-Hertani’s code and uploaded here: https://github.com/timknapen/Raspberry/tree/master/mmapGPIO
It works on my Pi2
did anyone evaluated the VNC/RDP performances changes.
My son (12 years) complained about the B+ oveclocked to super was somehow sluggish.
[…] Le code est disponible ici. […]
[…] hammers all four cores in parallel to see how the Pi 2 copes. (Splendidly, is the answer.) You can download the test he used to try it out on your own Pi […]
Results of ODROID-C1:
python Pi2test.py
How many threads?
>1
Thread: 0
Starting now…
Finished. That took 4.78 seconds
Thread 0 took 5.25 seconds
python Pi2test.py
How many threads?
>4
Thread: 0
Thread: 1
Thread: 2
Thread: 3
Starting now…
Starting now…
Starting now…
Starting now…
Finished. That took 4.97 seconds
Finished. That took 4.96 seconds
Finished. That took 4.98 seconds
Finished. That took 5.36 seconds
Thread 1 took 5.54 seconds
Thread 0 took 5.57 seconds
Thread 3 took 5.88 seconds
Thread 2 took 5.88 seconds
I was discusing the C1 with Daniel Bull last night and he said on paper the ARM should be about 30% faster. Looks like your stats seem to agree with that. He was chuffed to be right.
I did look at buying one, but the cheapest UK source I found would have been £38 delivered (which is about 30% more expensive).
I did this purely “for a laugh” as obviously this XU3 is not competing with the Pi due to it being more expensive. But anyway, the results from a $99 (including power supply and case) Odroid XU3Lite:
# python Pi2test.py
How many threads?
>1
Thread: 0
Starting now…
Finished. That took 2.05 seconds
Thread 0 took 2.28 seconds
# python Pi2test.py
How many threads?
>4
Thread: 0
Thread: 1
Thread: 2
Thread: 3
Starting now…
—-SNIP————
Finished. That took 2.30 seconds
Finished. That took 2.29 seconds
Finished. That took 2.38 seconds
Finished. That took 2.41 seconds
Thread 1 took 2.59 seconds
Thread 3 took 2.58 seconds
Thread 0 took 2.69 seconds
Thread 2 took 2.70 seconds
python Pi2test.py
How many threads?
>8
Thread: 0
Thread: 1
Thread: 2
Thread: 3
Thread: 4
Thread: 5
Thread: 6
Thread: 7
Starting now…
—-SNIP————
Finished. That took 2.81 seconds
Finished. That took 3.34 seconds
Finished. That took 3.47 seconds
Thread 5 took 3.72 seconds
Finished. That took 3.81 seconds
Finished. That took 4.00 seconds
Thread 4 took 4.13 seconds
Finished. That took 3.96 seconds
Finished. That took 4.07 seconds
Finished. That took 4.12 seconds
Thread 6 took 4.32 seconds
Thread 7 took 4.38 seconds
Thread 3 took 4.43 seconds
Thread 2 took 4.45 seconds
Thread 0 took 4.45 seconds
Thread 1 took 4.45 seconds
What HAVE I started? ;p
That’s pretty snappy, even if it’s 3 times the price.
XU3 appears to be $179.00 plus taxes plus shipping. How many PI 2s could we cluster into a bramble for that price? :-)
Was that the XU3 or XU3Lite Alex?
I benchmarked the XU3Lite which is the version without the Display Port and very slightly slower clock.
It is Python… C should be a *lot* faster.
A lot faster to run, but a lot slower to write in the first place ;-)
LOL – I was trying to think of a humourous way to explain the point of the comparison, but couldn’t find one, so gave up. ;)
C would certainly take longer to write in my case on account of not knowing C (apart from some arduino coding, which I’m told closely resembles C++)
[…] some good benchmarks. LinuxVoice has a nice overview with some performance tests. Alex Eames made nice video with power and performance measurements. On Hackady you can also see some nice […]
You might also try
$ sysbench –test=cpu –num-threads=4 –cpu-max-prime=20000 run
for a better multicore benchmark.
Sysbench is used in recent “rasp pi 2 vs other” benchmarks like here:
http://www.canox.net/2015/02/raspberry-pi-2-vs-banana-pi/
http://www.davidhunt.ie/raspberry-pi-2-benchmarked/
i5-4570 sysbench benchmark:
$ sysbench –test=cpu –num-threads=4 –cpu-max-prime=20000 run
sysbench 0.4.12: multi-threaded system evaluation benchmark
Running the test with following options:
Number of threads: 4
Doing CPU performance benchmark
Threads started!
Done.
Maximum prime number checked in CPU test: 20000
Test execution summary:
total time: 6.0464s
total number of events: 10000
total time taken by event execution: 24.1790
per-request statistics:
min: 2.30ms
avg: 2.42ms
max: 14.49ms
approx. 95 percentile: 2.57ms
Threads fairness:
events (avg/stddev): 2500.0000/43.93
execution time (avg/stddev): 6.0448/0.00
$
http://www.hardkernel.com/main/products/prdt_info.php?g_code=G140448267127
It get 50313 in Antutu.
https://drive.google.com/open?id=0B0MKgCbUM0itUnJYcVk3Z01zckE&authuser=0
My Nexus 5 (take in mind that its not running as much as it can because of cooling)
Ordroid is sh**. Expensive, problems with Exynos documenation and so on.
The Nexus 5 uses the Qualcomm SnapdragonTM 800. I had a look around and the only board that seems to have one of those is the DragonBoard 800 which is $500 so I’m not quite sure why you are claiming the Odroid is expensive since it scores roughly the same and is a fifth of the price?
Surely that makes it a bargain not expensive?
With reference to “problems with Exynos documentation” the Odroid supports multiple Linux distributions including Ubuntu, Android, and most mainstream apps and server applications such as XBMC, Apache, MySQL, Samba, etc.
Whilst I agree the Odroid community can’t match the Pis its actually pretty darn good and most definitley larger than most other boards coming in at least second or third place to the Pi.
I would like to use some of these data in a book, could you please contact me.
I’m happy for you to do that add long as you credit me as the source and put a link to RasPi.TV
I will do that, thank you very much for your kind permission.
[…] calcul est assez simple d’après le site raspi.tv le raspberry pi 2 consomme en pleine charge 350ma en 5V […]
Hi,
I have just a simple question. I have a pi 2 model B only with LAN cable (and nothing else). So, can I use a power supply with 850 mA?
Are you agree?
Should be OK as long as it really can deliver 850 mA and you don’t plug in lots of power-hungry USB devices :)
Is there any way to see how much faster that awesome gpu would do the same task?
I would imagine that the additional running cost is a small price to pay for all that extra processing power.
I want to know how you measurement the current drawn per core. what pins of RPi did you use to do the measurement and what was the hardware setup? I’m using RPi model B+ version2. I want to measure the change in power consumption on change in computational load. For that, I want to begin with measureing power consumption for any computional load.
I measure the current going directly into the Pi with a special device called an eMeter. See here for a video of how I do it…
https://raspi.tv/2014/raspberry-pi-a-how-much-power-does-it-need
Interesting, just to compare to the Raspberry Pi, I tried running this on a Odroid-XU:
odroid@odroidxu:~/temp/pi2test$ ./Pi2test.py
How many threads?
>1
Thread: 0
Starting now…
Finished. That took 2.30 seconds
Thread 0 took 2.55 seconds
odroid@odroidxu:~/temp/pi2test$ ./Pi2test.py
How many threads?
>4
Thread: 0
Thread: 1
Thread: 2
Thread: 3
Starting now…
Starting now…
Starting now…
Starting now…
Finished. That took 2.43 seconds
Finished. That took 2.45 seconds
Thread 0 took 2.92 seconds
Thread 3 took 2.88 seconds
Finished. That took 3.03 seconds
Finished. That took 3.03 seconds
Thread 2 took 3.45 seconds
Thread 1 took 3.47 seconds
It would be great to get more pieces of information about the power usage when doing GPU computation between the models.
Wouldn’t the GPU-power-usage be identical between models, since every model of RaspberryPi (including the B+, Pi2 and Zero) is still using the some VideoCoreIV GPU?
Good point. You’d think so. Might be a difference between the pre B+ models and the rest though because of the different power circuitry
That’s what the shooting video and redering video tests are there for in my standard batch of tests. Designed to exercise the GPU. If you meant something like using the GPU for FFT etc, well then you’d need someone cleverer than me to do it because I haven’t got a clue about that stuff :)
Thank you for your answer! I think the case can be extended into FFT quite easily. Do FFT computation in time and record it in video. Since it is video, rendering test should also be applicable.
It would be great if you could add accelerated FFT computation by GPU in the preliminary stage before the video recording. I am following your blog to see when you if you can do it. A thread about the power management between different models here http://raspberrypi.stackexchange.com/q/40905/32809. Accelerating FFT here https://www.raspberrypi.org/blog/accelerating-fourier-transforms-using-the-gpu/ by the founder of the Raspberry Pi. The code should be solid.
I think you’ll find it wasn’t Eben that wrote that FFT, but he may have written the post about it. Not that it matters much. As I said before, it lies outside my area of expertise, which means there isn’t much chance of me doing it in my standard tests.
Like Andrew said though, the GPU is the same on all Pi’s so far (Jan 2016). There may be small differences due to different efficiency of differing power circuitry, but the amount actually consumed by the GPU should be the same for all.
Is my understanding correct that you can reliably run the Pi2 under Raspbian & Python with 3 of the 4 cores shut down, so saving 140mA / 700mW ((0.420-0.280) x5V) saving by using Pi2 in single-core mode ?
I guess Pi2 must have a system setting parameter (perhaps similar to ‘arm_freq, gpu_freq, over_voltage’ etc in config.txt referenced in Version 1 of the User Manual) to turn on/off specific cores ?
I do not know Python (or Linux) so not clear to me how you knew the 3 cores were switched off, other than by deduction from measuring reduced power consumption.
I am interested to know what is a MINIMUM POWER CONSUMPTION in a light processing BATTERY powered environment (sensor logging) in a HEADLESS configuration, no USB connections. Your Power Results table states 230mA (1.15W) with ‘0 cores’ Also read elsewhere default ‘idle’ is 1.15W – does that mean 4-cores running-but-idle, or does Debian Power Management auto switch off 3 cores in idle ? (I could find nothing on Pi Power Management on the raspberry site)
Last question: any chance of you measuring Pi2 consumption with the ‘overclocking/overvolting’ parameters specified in the old (2012) User Manual (assuming they still work in hardware released after manual was written), i.e ‘arm_freq, gpu_freq, over_voltage’ etc set to their minimum (‘-16’ in config.txt) allowable to maintain stability. ?
“I am interested to know what is a MINIMUM POWER CONSUMPTION…”
In that case you really ought to be looking at using the Raspberry Pi model A+ or the Raspberry Pi Zero. https://raspi.tv/2015/raspberry-pi-zero-power-measurements
And to answer your other questions, I believe the cores are automatically “switched on and off” as needed by the Linux kernel, depending on CPU-load / how many threads are running. (Although AIUI the cores don’t get “switched off” when not needed, they simply “don’t get used” and/or “run at a slower clockspeed”). https://duckduckgo.com/?q=cpu+governer+raspberry+pi&t=canonical might be able to help you find some more info.
Thanks for what looks to be a very useful search criteria on subject of core switching which I will follow up asap as I am on the move just now.
As you are suggesting I pick a different Pi I better explain why I ask these questions about the Pi2 in hope they will not be ignored.
I fully understand there are SBCs more suited to sensor monitoring than any Pi with the necessary ADCs, RTCs that Pi’s don’t have, consuming far less energy than even a Pi-Zero. I realize at full blast, a Pi2 is overkill on processing power. However, my interest in the near-latest Pi, is
a) because I read it has far better community support than similar products from China, and
b) rather than pick a different horse for each job, I would rather choose a SBC that can hopefully be re-purposed over a wide range of tasks from HTPC (which the Pi2 is a bit weak on compared to the new quad A53 ARMv8 based SBCs due in weeks) down to the battery operated v. low power sensor logging I was hoping to use a Pi2 for.
I also realise default Raspbian, wastes power in having many running processes supporting a ‘Desktop environment’ not needed in a headless configuration. For that reason, I might discover a Pi supported OS (maybe one of the new IoT OS) more suited to low power sensor monitoring, that for example, puts the SoC to sleep until a time interrupt to process a logging event.
While researching the possibility of using the Pi2 in v. low power environments like IoT, as mentioned, I found nothing on Pi Power Management on the raspberry site. But I did find an interesting old article in MagPi Iss.23 5/2014 Page6: ‘Pi1b draws between 310 and 390mA in normal operation; this includes current draw of the HDMI output chip & the USB/Ethernet chips which account for nearly half of the total current. To prolong battery life during flight, these chips must be disabled. They are disabled by calling 2 Linux cmds. The C code to shut down the HDMI chip is:
system(“/opt/vc/bin/tvservice -off”); . Next cmd suspends the USB/Ethernet controller:
system(“sh -c \”echo 1 > /sys/devices/platform/bcm2708/usb/bussuspend\” “);
After disabling, avg current drops to 180mA. ‘ So if avg of 310-390 i.e 350mA normally, then the saving is 170mA. As the Pi2b has 4, not 2 USB, than saving may be higher.
I also read in some Cambridge Uni article, the Pi2 SoC boots at 600MHz, and by instruction only switches to 900MHz towards end of the boot. All of the above lead me to think it should be possible to get the Pi2 consumption down a lot when the HDMI and USB circuitry switched off, and hopefully able to run Raspbian on single A7 core. To ovoid making my initial post overly complex I left out this last info.
I was hoping Alex could re-run his measurement on the Pi2 using the minimum underclocking/undervolting parameter settings in config.txt that still allow his Python program to run reliably. The result of that – or even the news that those parameters (published in 2012) no longer work in the Pi2, would be helpful in deciding to pursue use of a Pi2 for battery powered sensor logging. If the current Pi2 design has easy setup parameters to switch off HDMI and USB, then interesting to know what power reduction it will achieve.
I agree that the Raspberry Pi has much better support (both from the community and the paid engineers at RPF) than any other similar board, and I can understand only wanting to use a “single horse solution”. However one of the fantastic things about the Pi (thanks to the wonderful work done by the RPF engineers) is that the same OS and SD card can be used to boot *any* model of RPi! So in your example you could do all your development on a Pi2, and then when you’re ready for your “battery-powered deployment” you can just switch the same MicroSD card into an A+ or Zero, and it’ll work just the same (using less power, albeit a little slower). The header-pins on the Pi2 / B+ / A+ / Zero are all identical too.
And the RPis are so cheap, there’s not much damage to your wallet in ordering more than one :)
If you want a “minimal” OS that doesn’t waste resources on running a desktop, then have a look at Raspbian Jessie Lite https://www.raspberrypi.org/downloads/raspbian/
If you want the Pi to be put to sleep until a timer interrupt, then you’ll need to use an external power-management board, e.g. http://spellfoundry.com/products/sleepy-pi/ or http://www.uugear.com/product/witty-pi-realtime-clock-and-power-management-for-raspberry-pi/ or https://pi.gate.ac.uk/pages/mopi.html or https://www.pi-supply.com/product/pi-ups-uninterrupted-power-supply-raspberry-pi/ or https://www.pi-supply.com/product/ups-pico-uninterruptible-power-supply-hat/ etc. etc. I’ve never used any of those boards myself, so have no idea which would be best suited for your application!
“As the Pi2b has 4, not 2 USB, than saving may be higher.”
The “bussuspend” power saving comes from switching off the LAN9512 chip (LAN9514 on the B+ and Pi2) which handles the USB and ethernet ports, and I *suspect* that the LAN9512 and LAN9514 have similar power consumption.
I suspect Alex is far too busy to run any extra power-management-requests from the community, so if you want that info you’ll probably need to run your own measurements. But AFAIK yes, those same 2 commands should still work on the Pi2. I certainly know that I’ve seen other users mention that they’ve been able to switch off the PiZero’s HDMI output for even lower power-usage.
You may be able to get additional help from http://www.raspberrypi.org/forums/ – lots more Pi experts there, than read the comments-section on Alex’s blog! ;-)
P.S. I haven’t measured if it reduces the power (or by how much) but I’ve just done a bit of ‘poking’ and it looks like the command to disable the LAN9514 on the Pi2 _might_ be:
echo 0 | sudo tee /sys/devices/platform/soc/3f980000.usb/buspower
(it looks a bit different to the previous command, due to the Pi’s kernel now using DeviceTree, so some of the /sys/ entries will have moved around)
After running that command, I see the following in the ‘dmesg’ output:
[ 171.377367] ERROR::dwc_otg_hcd_urb_enqueue:505: Not connected
[ 171.377367]
[ 171.513487] usb 1-1: USB disconnect, device number 2
[ 171.513515] usb 1-1.1: USB disconnect, device number 3
[ 171.513781] smsc95xx 1-1.1:1.0 eth0: unregister ‘smsc95xx’ usb-3f980000.usb-1.1, smsc95xx USB 2.0 Ethernet
[ 171.513849] smsc95xx 1-1.1:1.0 eth0: hardware isn’t capable of remote wakeup
which suggests that as far as the Pi is concerned, the USB and Ethernet ports have ‘disappeared’, and ‘ifconfig’ no longer shows any ‘eth0’ entry, and ‘lsusb’ just says:
Bus 001 Device 001: ID 1d6b:0002 Linux Foundation 2.0 root hub
I was testing this with a serial connection, as obviously doing the above from a USB keyboard, would then cause the keyboard to stop working ;)
Thank you for the information on a command line method of disabling the USB/Ethernet to reduce consumption and taking time to confirm it still works on the newer RPi2. Do you also know a commandline method to disable the HDMI chip ?
Thanks also for links to external power management hardware, whose feature lists remind me of warnings in older Pi documentation about memory corruption in event of unattended power-loss. eg. ‘Sleepy Pi’ (which at £30.83, even though adding my required RTC, may exceed my budget for my sensor logging idea) states ‘Avoid SD Card corruption with proper shutdown’. Reading further: http://spellfoundry.com/sleepy-pi/getting-sleepy-pi-shutdown-raspberry-pi/ I see a quite involved process to achieve safe shutdown in event of low power. I assume the ext hardware detects when after losing mainspower, battery power is about to expire and sets a pin low onthe Pi to run code to manage shutdown, after which Sleepy Pi removes power to the Pi. I read somewhere Pi’s have a dedicated reset pin to initiate a safe shut-down without need for a “sudo shutdown – h now”. Will that not achieve the desired result ? Once the Pi has ‘shutdown’, (as indicated by GPIO-14 Low) does that not mean its consumption has been reduced to some ‘Sleep’ spec ?
I have not had chance to read/digest all of the interesting indepth Mopi article you linked to but note their statement in 2013 “lots of unanticipated power cuts — a good way to corrupt your SD card… :-(” and Dave Akerman has run a Pi A? on only 3.3V to extend battery life. But with all this pre- Pi2, don’t know if corrupted SD during unplanned shutdown and ability to run Pi2 off 3.3V still applies. Unlike the Sleepy Pi which depowers the Pi completely when ‘not-needed’, I think the Mopi just provides automatic power switching from 2 sources; as such, the Sleepy does more for the money. Maybe the £18 UPS Pico is best fit, but wary as can find no documentation beyond a Feature List !
Do you know if there is a software method of preventing SDcard corruption during unplanned power-cuts, so the Pi2 can successfully resume sensor monitoring when power restored ?
If so, then the need for longterm battery backup and reduction of consumption is less important.
Re. my original question on reduction light processing current consumptionfrom (i.e idle) from the published 1.15W using the default voltage & clock speeds, by applying all the undervolting (0.4V) and underclocking parameters to the config.txt, and also disabling upto 3 cores without reducing ability to run Raspbian – you suggest I measure it myself. At this stage I am just considering the feasibility of using a RPi2 for the range of use-cases from HTPC to low-power battery operated sensor logger, as opposed to a SBC more suited to the latter. On a very practical level, I note that Alex used some expensive measurement kit: Alex post 12/11/14 states he uses a ‘eMeter’ in series with a benchtop psu set to 5.2V
Alex post ‘Review ISO-TECH IPS 3303D DC benchtop power supply’ states it cost £375 + VAT. Like your reply on 23/7/13 way out of my price range, without consideration of the eMeter !
The latter BTW appears quite an obscure product : http://media.hyperion.hk/dn/pc/doc/EmeterManualv1-28.pdf made I think around 2005, since replaced by V2 intended to measure very high currents with a coarser resolution of only 0.1 amps, so U/S for measuring 10’s or 100’s of mA. Reason Alex set the PSU to 5.2V was to cover the eMeter volt drop. All I have is a cheap multimeter, so IF the Pi2 is ok on 4.8V ??, I guess I could rig up a USB breakout with shunt resistor and measure the voltage across that to determine the current.
I will not be in the UK to purchase the choosen components until later this year so there is little I can do before then than research which SBC & sensor parts etc to use.
As you also have not the equipment to make measurements to add to Alex’s tables, might you be able to at least determine indirectly the effect of say, config.text Under-Clockings on a Pi2 to see if it affects Raspbian stability, and where a suitable CPU Benchmark is run (as I would run headless, no point measuring graphics) – as the benchmark speed should drop in proportion to CPU / Memory clock Speed. I suppose one could ‘assume’ no need to redo test with 3 cores disabled, as Alex’s tests inherantly confirm the Pi2 can run Raspbian on single-core. Without being able to measure current, I guess only point in then applying the Undervolting parameters is to confirm if Raspbian still stable at 0.4V down.
Re. rpi forum, seemed like the logical place to start, so I did in fact register with them last November to ask how to disable unused SBC parts like HDMI and USB to reduce consumption for battery run light processing/sensor logging, but got only 1 reply not answering my question, stating Pi2 takes 5 WATTS ! – (over 4x higher than in Alex’s idle/light processing power, higher even than the 3W PEAK Power Rating in ‘rpi2-model-b-hardware-general-specifications’) and seemed surprised anyone would want to reduce it even though I mentioned the [22mA] Arduino Stalker to indicate what IoT battery friendly SBCs can take. I did repost clarifying my question to say still unanswered. Maybe I posted in the wrong section, but having heard nothing after a month I spent many mandays sifting through posts on other sites such as yours to discover what I think should be more easily accessible information. As stated in my initial post, I do not know Python or Linux so being a newbie, I need to research everything to decide if the RPi2 consumption can be reduced sufficiently to achieve my aims for sensor logging. As others pointed out on that forum, the Pi User Manual is badly in need of updating to include data on the newer Pi’s and the UI changes so newcomers do not lose so much time trying to find basic info. But to put in perspective, there is a huge mass of now partly outdated literature on Pi’s in general, whereas the many cheaper Chinese products appear to have almost no community support.
I think it would help the community to find answers faster if like in some other tech forums, there was provision for Topic Tagging and all posters encouraged to make full use of the tagging system. I think articles in MagPi and other magazines on the Pi family should introduce at least a minimal tagging system, 1 colour or icon for each Pi model, to indicate for each article, if it applied only to eg. Pi2, PiZero, Pi1A etc. That can quickly eliminate confusion over which articles are relevant.
My E-meter has a calibrated 20 Amp shunt which measures two decimal places of an amp. There is no significant voltage drop across it. That’s one of the main reasons I use it. As standard it came with a 100 Amp shunt, but I bought the extra 20 Amp shunt for greater accuracy at the low end. Since my readings usually match the officially released figures quite closely, and the shunt is calibrated, I trust them to within 10 or 20 mA.
I wouldn’t have bought a £375 bench PSU either, but when offered one in exchange for a review video (on which I spent about 20 hours) I would have been positively foolish to refuse. It’s a lovely piece of kit.
Wow, was that a new record for your longest blog comment Alex? ;-)
“Do you also know a commandline method to disable the HDMI chip ?”
I believe you can still use the “tvservice -off” command that you posted earlier to disable the HDMI-output portion of the SoC.
“I read somewhere Pi’s have a dedicated reset pin to initiate a safe shut-down”
Nope, the reset pin on the Pis is a hardware-level reset, i.e. it does an “unsafe” hard-reboot (the same as removing and then inserting the power cable).
“does that not mean its consumption has been reduced to some ‘Sleep’ spec ?”
I’ve got no idea, that’s something you’ll need to test for yourself. But as the Raspberry Pi has no deep-sleep power-management circuitry that you might be used to on a microcontroller, then I wouldn’t be surprised if the ‘Sleep’ power-consumption was similar to the ‘Idle’ power consumption. We Just Don’t Know!
“don’t know if corrupted SD during unplanned shutdown”
Unplanned shutdown *always* has a risk of causing SD-card corruption – just like you can’t just yank the power-lead out of a desktop PC but must always do “Shutdown” first.
“ability to run Pi2 off 3.3V still applies”
I’ve got no idea, but with the higher power-demands (and different power-circuitry) of a Pi2 that *may* not be possible.
But as I said in an earlier comment, the Pi 2 and Pi A+ are fully software-compatible, so I’ve got no idea why you’re so insistent on trying to reduce the power-consumption of a Pi 2, rather than simply using an A+. (and I suspect this may also be why you didn’t get much help on the RPi forums)
“Do you know if there is a software method of preventing SDcard corruption during unplanned power-cuts”
The only way to do that is to mount the filesystem on your SDcard read-only. But of course that’s then not much use for sensor logging because you can’t save any data… but see my comment here too https://raspi.tv/2013/pi-duration-tests-and-review-of-two-new-lithium-battery-packs#comment-32330
Obviously to minimise the risk of corruption, you want as few things writing to the card as possible, so if you don’t have a “safe shutdown system” set up, you probably want to be running a very stripped-down system with minimal background services etc.
But even then if you’re very unlucky the power to the Pi *might* cut out at exactly the same time as you’re writing data to the SD card.
“so IF the Pi2 is ok on 4.8V ??”
I believe the “official spec” is 5V +/- 5% i.e. 4.75V to 5.25V
“might you be able to at least determine indirectly the effect of say, config.text Under-Clockings on a Pi2 to see if it affects Raspbian stability”
No. I’ve already spent lots of time replying to your comments, I’m not going to waste time messing about with underclocking and stability tests.
“I mentioned the [22mA] Arduino Stalker to indicate what IoT battery friendly SBCs can take”
No model of Pi will ever get down to those kinds of power levels – if you really want something so low-power then you definitely need to use a microcontroller. But of course a microcontroller in turn can’t run a full Linux system, it’s the old “horses for courses” / “picking the right tool for the job”…
But (some) microcontrollers *can* run Python http://micropython.org/
Obviously when the older articles were being written for old issues of MagPi, the Pi2 and PiZero didn’t exist yet, so the authors didn’t know that what they were writing wouldn’t be compatible with newer models of Raspberry Pi ;-)
I agree that there’s now lots of old outdated literature on the internet that was written for older models of Raspberry Pi (and older versions of Raspbian), but there’s obviously nothing that can be done about that. The only way to tell which info is ‘outdated’ and which is ‘current’ is to use your best judgement, and that’s obviously something that’s very difficult for somebody new to the Raspberry Pi! (but I guess looking at the dates that the articles were published might give you a clue)
But I believe all the hard-working people at the RPF (with help from volunteer contributors) do their best to keep the “official documentation” at https://www.raspberrypi.org/documentation/ up to date.
There is no HDMI chip. That would be the Broadcom SOC, if you disable that you have no Raspberry Pi.