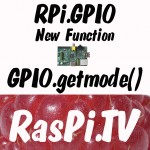
Another new RPi.GPIO feature that I discovered last week is GPIO.getmode(). This appeared in RPi.GPIO 0.5.11 and allows you to query RPi.GPIO to see whether GPIO.setmode() has been set up as BCM, BOARD, or UNSET mode. This could be useful if you are running a suite of scripts or modules which work together. GPIO.getmode() returns…
-1 if GPIO.setmode() is not set
11 if GPIO.setmode(GPIO.BCM) is active
10 if GPIO.setmode(GPIO.BOARD) is active
Below you can see a live python session showing what you get when you use GPIO.getmode() with different modes set…
Why Did You Bother Setting Up An Input?
Good question. Glad you asked. Once you’ve set the mode, you can only change it once you’ve done a GPIO.cleanup(). But you can only do GPIO.cleanup() once you’ve configured a port. So you can’t flick between GPIO modes without first setting up a port, then cleaning up. Apart from testing, as I’m doing here, it’s most unlikely anyone would actually want to do that. So it’s probably a bit of a non-issue. I always use BCM numbering, so wouldn’t need to do that.
Let’s have a closer look at that live Python session to see what’s happening…
>>> from RPi import GPIO # bring in the needed library >>> mode = GPIO.getmode() # read the mode into variable >>> print mode # print the value -1 # -1 is the output >>> GPIO.setmode(GPIO.BCM) # set BCM mode >>> mode = GPIO.getmode() # read the mode into variable >>> print mode # print the value 11 # 11 is the output
Now we need to configure an input port because we can’t switch between modes without using cleanup(), which won’t work unless we’ve configured a port.
>>> GPIO.setup(24, GPIO.IN) # set up an input >>> GPIO.cleanup() # clean up >>> GPIO.setmode(GPIO.BOARD) # set BOARD mode >>> mode = GPIO.getmode() # read the mode into variable >>> print mode # print the value 10 # 10 is the output
How About A Little Script?
Let’s write a little script that we can use to show all of this in action.
from RPi import GPIO from time import sleep modes = {-1:"Unset", 11:"BCM", 10:"BOARD"} mode = GPIO.getmode() print("Your Pi is in ", modes[mode], " mode") sleep(3) print("Changing to BCM mode") GPIO.setmode(GPIO.BCM) mode = GPIO.getmode() print("Your Pi is now in ", modes[mode], " mode") sleep(3) GPIO.setup(24, GPIO.IN) # configure a port so can cleanup GPIO.cleanup() # cleanup so can set mode again print("Changing to BOARD mode") GPIO.setmode(GPIO.BOARD) mode = GPIO.getmode() print("Your Pi is now in ", modes[mode], " mode") sleep(3) print("Exiting - we're done!")
The output from this script looks like this…
It may not be something you’ll use every day – or even at all. But it’s good to know it’s there in case you need it. I include it for completeness as I like to document all the features of RPi.GPIO.
RasPiO® GPIO Reference Aids
Our sister site RasPiO has three really useful reference products for Raspberry Pi GPIO work...
Looking at http://sourceforge.net/p/raspberry-gpio-python/code/ci/ab4fbdffb322cebaaef35d406b948af67ef152f4/tree/test/test.py?diff=8c1497ab34260214c231fa021d3f01dfd7c05ed8 (which is part of the changes made when getmode() was added) it looks like you can just use the constants GPIO.UNKNOWN, GPIO.BCM and GPIO.BOARD rather than the hard-coded values -1, 11 and 10; which is both more flexible and IMHO much easier to read :-)
Could you show us an example of how you’d use that Andrew? I don’t quite understand.
Sorry, perhaps that wasn’t the best link to use. This might be easier to read http://sourceforge.net/p/raspberry-gpio-python/code/ci/default/tree/test/test.py#l70
It means you could e.g. change your ‘modes’ dictionary to:
modes = {GPIO.UNKNOWN:”Unset”, GPIO.BCM:”BCM”, GPIO.BOARD:”BOARD”}
i.e. GPIO.BCM (internally) has the value 11, and IMHO checking if mode == GPIO.BCM looks much neater than checking if mode == 11.
Yeah – that makes sense. Thanks. :) I saw the brief documentation mentioning those constants, but when I came to actually view the values of getmode() I got the numbers. I can now see how they relate. It wasn’t immediately obvious to me how to use GPIO.UNKNOWN etc. And if it wasn’t obvious to me, you can bet it wasn’t obvious to a whole load of other people.
I think this is likely to be a “useful to some but not used widely” feature. I like to try to document all the features though. (Pity I can’t fit more on the ruler – then again – maybe it’s not!)
You’ll just have to bring out a 12″ ruler as a sequel… ;-)