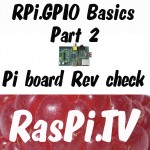
It all started in September 2012. The Raspberry Pi Foundation went and made some improvements to the Pi. How dare they!! ;) Seriously, though, there were some significant improvements to the Pi and a Rev 2 version was launched with double the RAM and some other changes/additions/improvements.
This threw up some minor headaches for developers because some of the GPIO pinouts were changed and some new GPIO ports were made available on a brand new “solder it yourself if you want it” header called P5 (see the leaning header of Pi5a)
GPIO 0 became GPIO 2 (P1 pin 3)
GPIO 1 became GPIO 3 (P1 pin 5)
GPIO 21 became GPIO 27 (P1 pin 13)
The two i2c buses were swapped around:
SDA0 became SDA1 (P1 pin 3 alternative function)
SCL0 became SCL1 (P1 pin 5 alternative function)
Some new GPIO ports 28, 29, 30, 31 were made available if you solder on P5 header.
(Update Dec 2014: We also have the Compute Module, the B+ and the A+ now, so it’s really useful to be able to check what sort of Pi a program is running on.)
Who cares?
People whose programs no longer work with all Raspberry Pis – that’s who!
For example, the Gertboard LEDs program uses GPIO21. If using a Rev 1 Pi this worked fine, but on a Rev 2 Pi (including model A) the fifth LED wouldn’t light because GPIO21 is now GPIO27. To work properly, the program needs to be able to know whether it’s running on a Rev 1 or Rev 2 Pi, so it can use the correct ports.
How to check your Raspberry Pi Revision number?
There’s a way to see what your Pi Revision is…
cat /proc/cpuinfo
You can see this gives lots of info. Near the bottom is “Revision : 000f”
This tells me I have a Rev 2 Pi, but it’s a bit clunky. There are several different rev. codes for different Pi models and manufacturers (here’s a list of them). We could write some code to check the cpuinfo and extract the bit we want, compare it with known revision codes etc. But we don’t need any of that because, from RPi.GPIO 0.4.0a onwards (September 2012) we can use a built-in RPi.GPIO variable which does it all for us. (If you have older than 0.4.0a, see yesterday’s post for updating instructions.)
It’s called GPIO.RPI_REVISION
As before, we can try this out in a live python session…
sudo python
import RPi.GPIO as GPIO
GPIO.RPI_REVISION
Mine returns a 2 because it’s a Rev 2 Pi.
Possible answers are 0 = Compute Module, 1 = Rev 1, 2 = Rev 2, 3 = Model B+/A+
Or you can incorporate the code into a program as we did in yesterday’s post …
import RPi.GPIO as GPIO print "Your Pi is a Revision %s, so port 21 becomes port 27 etc..." % GPIO.RPI_REVISION
So then, when you’re doing some GPIO work that uses any of the ports 0, 1, 2, 3, 21, 27, 28, 29, 30, 31, you can be sure to control the correct ports. See the example below…
import RPi.GPIO as GPIO if GPIO.RPI_REVISION == 1: ports = [0, 1, 21] else: ports = [2, 3, 27] print "Your Pi is a Revision %s, so your ports are: %s" % (GPIO.RPI_REVISION, ports)
As long as no further changes to GPIO pin allocations are made, the above should work with all future Pi revisions. If further changes are made, they will need to be incorporated like this (and Ben will have to update RPi.GPIO again)…
import RPi.GPIO as GPIO if GPIO.RPI_REVISION == 1: ports = [0, 1, 21] elif GPIO.RPI_REVISION == 2: ports = [2, 3, 27] else: ports = ["whatever the new changes will be"] print "Your Pi is a Revision %s, so your ports are: %s" % (GPIO.RPI_REVISION, ports)
What next?
That was part 2: checking your Pi board revision number. Tomorrow, in part 3 we’ll cover the best way to handle a clean CTRL+C exit, protect your Pi and avoid all those nasty, horrid, “port already in use by another program” messages.
RasPiO® GPIO Reference Aids
Our sister site RasPiO has three really useful reference products for Raspberry Pi GPIO work...
[…] the next part, we’ll cover how to check what revision of Raspberry Pi you have. Currently there’s only Rev 1 and Rev 2, but there are some GPIO differences (e.g. i2c and […]
If you use the BOARD numbering system rather than the BCM numbering system then the differences in board revisions are handled automatically for you. Your code will not need to detect board revision itself.
Yes indeed. I forgot to mention that because I habitually use the GPIO numbering scheme. Thanks for adding that Ben :)
Oh yeah – and because I’m doing this “backwards” I won’t cover that until day 4 :)
p.s. Does the board scheme cover the P5 header on Rev 2 Pis?
I’ve just had a look at the source code ( http://code.google.com/p/raspberry-gpio-python/source/browse/source/common.c ) and the BOARD numbering scheme only covers the 26 pins on the P1 header. In order to access the GPIOs offered by the P5 header you need to switch to the BCM numbering scheme.
Ben – I wonder if it’d be worth making BOARD_P1 a synonym of BOARD, and then adding a corresponding BOARD_P5 numbering scheme?
I didn’t think it did. I remember when I did the “Pi5a” header, I think someone asked in a Python thread on the Pi forums if you could use the BOARD numbering scheme for this. It only worked with GPIO BCM numbering.
It’s great to have a choice, but it seems sensible to me for people to pick one system and stick to it. I use GPIO BCM numbering because I got started GPIO programming when I first bought a Gertboard, and that’s what Gertboard uses.
Even if you use BOARD numbering, you still have to be aware of what the pins are, so you don’t end up connecting a button between e.g. GND and 5V
Yeah, that’s where the Raspberry Leafs come in handy :-) http://www.raspberrypi.org/archives/3307
https://docs.google.com/file/d/0B23BvTk93HRYOXVwbjVrWHRjbDg/edit?usp=sharing is a better version than the original IMHO.
Getting the revision is useful even if using board numbering (P1/P5 connectors numbering) as you may also want to access I2C and that chamged at same time.
Personally using Board numbering makes circuit/wiring instructions match the software and easier to follow especially for beginners
For anybody curious, you can find a list of the different
/proc/cpuinfo
revision codes at http://elinux.org/RPi_HardwareHistoryAnd if you are not wedded to Python, there are a handy bunch of scripts and code examples for finding board revision in several languages at http://raspberryalphaomega.org.uk/2013/02/06/automatic-raspberry-pi-board-revision-detection-model-a-b1-and-b2/
I’m confused. I tried both methods and get different results, i.e. dumping /proc and I see a “0002”, but running the python code, I’m told “1”. Ideas? Thanks in advance. s.
stephe@srw-pi ~ $ cat /proc/cpuinfo
Processor : ARMv6-compatible processor rev 7 (v6l)
BogoMIPS : 697.95
Features : swp half thumb fastmult vfp edsp java tls
CPU implementer : 0x41
CPU architecture: 7
CPU variant : 0x0
CPU part : 0xb76
CPU revision : 7
Hardware : BCM2708
Revision : 0002
Serial : 00000000ce955231
stephe@srw-pi ~ $ sudo python
Python 2.7.3rc2 (default, May 6 2012, 20:02:25)
[GCC 4.6.3] on linux2
Type "help", "copyright", "credits" or "license" for more information.
>>> import RPi.GPIO as GPIO
>>> GPIO.RPI_REVISION
1
>>>
Easy answer:
0002 is one of the Rev 1 codes
AndrewS linked to a list of them 2 comments above. I think I’ll incorporate that link into the main post though.
Urg! Thanks. I’ll read the WHOLE post and links next time. s.
0002 and 0003 were the Rev 1 codes, but it was made a bit more complicated in that if you overvolted your Pi, the code became 10000002 or 10000003, so you had to pull the last four digits from the code to parse the value properly.
Everything else can still be treated as Rev 2 – until they bring out the next batch of changes, that is :)
[…] 2: “how to check what Pi board Revision you have” follows up […]
Whats about the Problem that GPIO21 is used from RaspiCam?
Hi! I have been reading your tutorials and I have a problem here. Note: I have a Raspberry Pi B+
When using cat /proc/cpuinfo I get this:
Hardware : BCM2708
Revision: 0010
then I used this:
sudo python
import RPi.GPIO as GPIO
GPIO.RPI_REVISION
and I got 2.
So the question is, if it is a Raspberry Pi model B+, shouldn´t the revision be 3 as you have said?
Is it possible that your raspbian install is old or your RPi.GPIO version is old?
I don´t know. I’m doing a traineeship in a company and I have to use it for a project. So I was researching a little bit about Raspberry Pi and how it works.
How do I know which raspbian is installed?
I have used this yesterday to know which version of the RPi.GPIO it has:
sudo python
>>> import RPi.GPIO as GPIO
>>> GPIO.VERSION
and I got a ‘0.5.5’
if I install the ‘0.5.11’ version, tha last one as I have seen, would the revision change?
I’m worried because I don´t want to do something that might crash the Raspberry Pi B+
sorry, I do know now which raspbian I’m using ( I used uname -a, I thought there was another option of doing so). What I got is this:
Linux raspberrypi 3.12.22+ PREEMPT Wed Jun 18 18:29:58 BST 2014 armv6l GNU/Linux
Easiest way to tell what version of Raspbian you have installed is:
cat /boot/issue.txt
If you installed RPi.GPIO via apt-get (which is the recommended way), then you can upgrade to the latest version by simply doing:
sudo apt-get update
sudo apt-get install python-rpi.gpio
(assuming your Pi is connected to the internet of course)
thanks for it, I tried it and got this:
Raspberry Pi reference 2014-06-20 (armhf)
Generated using spindle, http://asbradbury.org/projects/spindle/, 8fe9fd4, stage 4-lxde-edu.qed
About the RPi.GPIO I haven´t done anything yet with the Raspberry Pi. I wanted to research well before starting ‘playing’ with it.
You may be better off getting hold of a spare microSD card, and using the latest Raspbian version
https://www.raspberrypi.org/downloads/
That way you’ll have much more up-to-date versions of everything, and by doing it all on a separate card, there’s no risk of messing up the original card.
and the GPU (I don´t know if I do need it for something either):
/opt/vc/bin/vcgencmd version
and I got this:
Jun 18 2014 18:46:58
Copyright (c) 2012 Broadcom
version 1a6f79b82240693dcdb9347b33ab16f656b5f067 (clean) (release)
this is the info of the cpu:
using lscpu:
Architecture: armv6l
Byte Order: Litlle Endian
CPU(s): 1
On-line CPU(s) list: 0
Thread(s) per core: 1
Core(s) per socket: 1
Socket(s): 1
using cat /proc/cpuinfo
processor: 0
model name: ARMv6-compatible processor rev 7(v6l)
Features: swp half thumb fastmult vfp edsp java tls
CPU implementer: 0x41
CPU architecture: 7
CPU variant: 0x0
CPU part: 0xb76
CPU revision: 7
Hardware : BCM2708
Revision: 0010
Serial: 0000000028cdad09
what does ‘processor:0’ mean?
Sorry for the several posts and thank you for your help in advance
It means you only have 1 processor, at index 0.
On the Pi2 (which has a quad-core CPU), running
cat /proc/cpuinfo | grep processor
results in
processor : 0
processor : 1
processor : 2
processor : 3
Thanks both of you, AndrewS and alex,
After talking with the guys in the company I was allowed to update the RPi.GPIO. I did it, and now:
in GPIO.RPI_REVISION I got ‘3’.
Thanks!!
If you just want to know if its a PI or a PI2, you can also do a
cat /proc/cpuinfo | grep Hardware
this will result in
Hardware : BCM2708
(PI)
Hardware : BCM2709
(PI2)
I am using this technique to convert a GPIO library to use the appropriate memory addresses as they are different between the two processors.
This info seems to be a bit dated now. For the Raspberry 3 B, I don’t see any discussion of ports, just pins.
I’m going to ignore this part and see if the rest of it works…