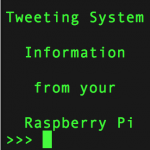
Today I’m going to show you how to tweet some system information from your Raspberry Pi. In part 2, we did a basic tweet entered at the command line, with a standard, fixed, default message if no tweet text was entered.
But That Default Text was Pretty Boring
So let’s do something more fun with it. Let’s make it tweet the time, date and processor temperature if you don’t enter any tweet text.
I got the ‘tweet the cpu temperature’ idea from Chris Mobberly’s blog. Here’s a condensed code snippet we’re going to borrow from Chris….
import os cmd = '/opt/vc/bin/vcgencmd measure_temp' line = os.popen(cmd).readline().strip() temp = line.split('=')[1].split("'")[0] tweet_text = 'My Current Processor Temperature: '+ temp + ' C' api.update_status(status=tweet_text)
We’ll mix it up with some of our own code from the previous script and add a bit of new.
You Can’t Tweet the Same Thing Twice in a Row
When I was messing around with Chris’ script, an issue arose when two successive tweets were of the same temperature value. Tweepy threw an error. It won’t let you tweet the exact same thing twice in a row. So if you run the program twice without any tweet text, and the temperature is the same, it won’t send the second tweet.
The way round this was to make each tweet unique by ‘time-stamping’ the tweets. Having got that working, I decided they needed the degree symbol too. So I retrieved the correct unicode character from a script I’d written in the past.
Here’s the Code
In lines 5-6 we’re importing some new libraries to handle the temperature query and time-stamping.
In line 8 we take a snapshot of the time and date
In line 9 we assign a variable with unicode character for the degree symbol
Lines 11-25 are the same as part 2
Lines 27-33 deal with reading the CPU temperature and tweeting it, if no tweet text is entered.
Lines 35-38 are the same as part 2
#!/usr/bin/env python2.7 # tweet2.py by Alex Eames https://raspi.tv/?p=5941 import tweepy import sys import os from datetime import datetime i = datetime.now() degree = unichr(176) # code for degree symbol # Consumer keys and access tokens, used for OAuth consumer_key = 'type in your consumer key here' consumer_secret = 'type in your consumer secret here' access_token = 'type in your access token here' access_token_secret = 'type in your access token secret here' # OAuth process, using the keys and tokens auth = tweepy.OAuthHandler(consumer_key, consumer_secret) auth.set_access_token(access_token, access_token_secret) # Creation of the actual interface, using authentication api = tweepy.API(auth) if len(sys.argv) >= 2: # use entered text as tweet tweet_text = sys.argv[1] else: # if no entered text, tweet the temp now = i.strftime('%Y/%m/%d %H:%M:%S') cmd = '/opt/vc/bin/vcgencmd measure_temp' line = os.popen(cmd).readline().strip() temp = line.split('=')[1].split("'")[0] print now + ' Pi Processor Temperature is '+ temp + ' ' + degree +'C' tweet_text = now + ' Pi Processor Temperature is '+ temp + ' ' + degree +'C' if len(tweet_text) <= 140: api.update_status(status=tweet_text) else: print "tweet not sent. Too long. 140 chars Max."
Program Output
The first time I ran it, it just tweeted the text I entered. (Remember to use quotes.)
The next time, with no entered text, it displayed and tweeted the full Date, Time and CPU temperature information.
What Can You Do With It?
We're now well on our way to integrating twitter into a hardware application, like a weather station. You could use this method to tweet any kind of input you can read with a Pi. The possibilities are almost limitless. It's really quite exciting.
But before we start on that side of things, there's one or two more useful tweepy technique I want to cover. Next in the series will cover how to add a photo to a tweet. This is where it starts to get really interesting.
Just a super minor point, but IMHO:
print now + ' Pi Processor Temperature is '+ temp + ' ' + degree +'C'
tweet_text = now + ' Pi Processor Temperature is '+ temp + ' ' + degree +'C'
is ‘nicer’ if re-written as:
tweet_text = now + ' Pi Processor Temperature is ' + temp + ' ' + degree +'C'
print tweet_text
You’re right (as usual) :)
The reason the print was there originally was for debug, but I ended up leaving it in.
Obviously for debug, you would need to see what you wanted to tweet before you tweeted it, hence the order. But you’re right, afterwards it can be much more elegantly done the way you suggest.
How easy should it be to set up a cronjob to run the script every 5 minutes?
I have been able to set up a cronjob that tweets however it only does it once, unless I remove all existing tweets from twitter.
unless I edit the cron job and add something to the then this will work
im very new to this so a simplified answer would be best.
Cheers
2 things you need to be aware of when using cron…
1. All file paths must be absolute. I learned this the hard way when my script would work fine when I ran it, but not from cron
2. Tweepy won’t allow you to tweet the same text twice in a row. It will block the duplicate tweet. This may be what you’re experiencing?
LOL. snap! I suspect it’s probably twitter that’s blocking the duplicate tweet, rather than Tweepy itself though?
Could be, but I think tweepy does have that feature.
Are you sure you’re not just seeing the “It won’t let you tweet the exact same thing twice in a row.” thing, that Alex mentions above?
When I run the python file with the same values to tweet more than once I do get the error saying its a duplicate.
Doing this through cron it doesnt come up with a duplicate tweet message.
what do you mean by absolute sorry?
my command for cron is */1 * * * * sudo python /home/pi/tweet.py
if I append “test” that works
if u change “test” to “test1” that runs from cron.
ive also tried running from crob manually tweet something before the next scheduled cron job amd this doesn’t work.
any suggestions would be great.
That’s why I put a time stamp in to guarantee that no two tweets will be identical, even if the other data is the same.
by absolute file path I mean the whole path to the file e.g. /home/pi/file.txt instead of just file.txt.
I think my scripts all use only absolute file paths, but if you’ve changed anything, it’s something you need to be aware of because cron runs as root user, you can’t assume it will start from /home/pi, so you have to specify it. I found that one out the hard way :)
Ive used absolute paths and kept the file in /home/pi/ directory for now.
Could I be using cron incorrectly?
I know you can do crontab -e
or specify user e.g. -u root or pi? Or sudo crontab.
Could this be the issue? I have been using the command crontab -e .
ive looked into crontab and notice there are cron.allow / deny but as the job does run im guessing permissions isnt going to be the problem
I’m not very expert with cron, but I did find it was quite fussy. Hope someone else can help you with that part.
I haven’t tried this myself (don’t have a twitter account) but from what you’ve written above it sounds like your cronjob is working absolutely fine, and it’s simply the duplicate tweet problem you’re running into?
Cronnjobs run as a “system process” so you won’t see any error messages – that’s to be expected. You’ll only see error messages if you run the script directly from a terminal.
Hi all just thought I would give an update to my initial query. I have finally managed to set up a cronjob to tweet every minute the temp.
AndrewS as you mention cron does not give out errors, after a search on the internet and trial and error, i have managed to get the cronjob to output errors.
the code i used was:
crontab -e
*/1 * * * * python /home/pi/tweet/tweet.py &&’date >> /tmp/errorlog.txt 2>&1
i could then make changes to the script as needed whilst using tail -f command to see any errors.
without the &&’date’ i was in fact running into a Duplicate tweet error.
with the duplicate error out of the way I then ran into a second error regarding the Degrees Character and not being able to encode it correctly.
to get around this I did the following
changed degree = unichar(176) to be degree = ‘o’
I then removed ‘+ degree ‘ from lines 32 & 33 ..
print now + ‘ Pi Processor Temperature is ‘+ temp + ‘ ‘ + degree +’C’
tweet_text = now + ‘ Pi Processor Temperature is ‘+ temp + ‘ ‘ + degree +’C’
This now works.
Thank you all for all your help
Hi, I’m using your script to send tweets which gets information from nagios.
However i’m trying to use a macro which is in epoch format.
Do you have any idea how i could convert the epoch format in your script?
Hmm, google might know? ;-)
https://www.google.co.uk/search?q=python+time+and+date+conversion