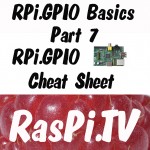
In the previous six articles, we’ve covered the basics of RPi.GPIO. I hope the series has been useful.
While I was doing the “recap” for part 6, I decided to make myself a quick reference “cheat sheet” with all the common RPi.GPIO stuff in it. I figured this would be useful and save me having to look things up on the web and in previous Python scripts I’ve written. I made it as a text file that I can just copy and paste snippets of code from to make RPi.GPIO Python coding easier.
Then I realised that it would make a perfect part 7 to round off the series – along with links to the more advanced RPi.GPIO tutorials on interrupts and PWM that I published back in April. So here it is…
RasPi.TV RPi.GPIO Quick Reference “cheat sheet”
I’ll put it here as a “Python script”, and there’s also a downloadable PDF with a full breakdown of all the P1 ports for both Rev 1 and Rev 2 Pis and a full list of links to all 12 RPi.GPIO tutorials on RasPi.TV.
There’s also a .txt version you can either download or wget straight onto your Pi.
wget https://raspi.tv/download/rpigpio.txt
# RPi.GPIO Basics cheat sheet - Don't try to run this. It'll fail! # Alex Eames https://raspi.tv # https://raspi.tv/?p=4320 # RPi.GPIO Official Documentation # http://sourceforge.net/p/raspberry-gpio-python/wiki/Home/ import RPi.GPIO as GPIO # import RPi.GPIO module # choose BOARD or BCM GPIO.setmode(GPIO.BCM) # BCM for GPIO numbering GPIO.setmode(GPIO.BOARD) # BOARD for P1 pin numbering # Set up Inputs GPIO.setup(port_or_pin, GPIO.IN) # set port/pin as an input GPIO.setup(port_or_pin, GPIO.IN, pull_up_down=GPIO.PUD_DOWN) # input with pull-down GPIO.setup(port_or_pin, GPIO.IN, pull_up_down=GPIO.PUD_UP) # input with pull-up # Set up Outputs GPIO.setup(port_or_pin, GPIO.OUT) # set port/pin as an output GPIO.setup(port_or_pin, GPIO.OUT, initial=1) # set initial value option (1 or 0) # Switch Outputs GPIO.output(port_or_pin, 1) # set an output port/pin value to 1/GPIO.HIGH/True GPIO.output(port_or_pin, 0) # set an output port/pin value to 0/GPIO.LOW/False # Read status of inputs OR outputs i = GPIO.input(port_or_pin) # read status of pin/port and assign to variable i if GPIO.input(port_or_pin): # use input status directly in program logic # Clean up on exit GPIO.cleanup() # What Raspberry Pi revision are we running? GPIO.RPI_REVISION # 0 = Compute Module, 1 = Rev 1, 2 = Rev 2, 3 = Model B+ # What version of RPi.GPIO are we running? GPIO.VERSION # What Python version are we running? import sys; sys.version
Links to more advanced RPi.GPIO tutorials
If this RPi.GPIO basics series has made you want to dig deeper into GPIO programming/physical computing, you’ll be glad to hear that there are some more advanced features available in RPi.GPIO.
Interrupts are a much more efficient way of handling “button press” type inputs than the “polling loop” we used in our basic example. There’s a series of three articles about interrupts.
Pulse-width Modulation (PWM) can be used to control things (e.g. motor speed) more precisely than just on and off. There’s a two-part series on that as well.
Interrupts (needs RPi.GPIO 0.5.2+)
- Background and simple interrupt: How to use interrupts with Python on the Raspberry Pi and RPi.GPIO
- Threaded callback: How to use interrupts with Python on the Raspberry Pi and RPi.GPIO – part 2
- Multiple threaded callback: How to use interrupts with Python on the Raspberry Pi and RPi.GPIO – part 3
- Edge Detection: Detecting both rising and falling edges with RPi.GPIO
Software PWM
From 0.5.2a onwards, RPi.GPIO has software PWM. Below are two links. One describes its basic usage and the other is a practical application for dimming LEDs and controlling motor speed.
- RPi.GPIO 0.5.2a now has software PWM – How to use it
- How to use soft PWM in RPi.GPIO 0.5.2a pt 2 – led dimming and motor speed control
I haven’t forgotten about the Darlington
In part 5, I mentioned a future article about the use of a Darlington array. That’s not strictly RPi.GPIO, and will come at some point, but if you’re desperately impatient about that you can click here to see a bit about Darlington arrays as part of the PWM tutorial.
Thanks Ben
No series on RPi.GPIO would be complete without a big thank you to RPi.GPIO author Ben Croston. Thank you for all your hard work on making it easy for us to control the world with GPIO on our Raspberry Pis. The official RPi.GPIO documentation is found here.
Download the cheat sheet
So, that’s about it for RPi.GPIO basics.
You can download the Quick Reference cheat sheet here.
And you can download the ‘cut and paste’ text version here. or wget https://raspi.tv/download/rpigpio.txt
Links to all the RPi.GPIO Basics series
- How to check what RPi.GPIO version you have
- How to check what Pi board Revision you have
- How to Exit GPIO programs cleanly, avoid warnings and protect your Pi
- Setting up RPi.GPIO, numbering systems and inputs
- Setting up and using outputs with RPi.GPIO
- Using inputs and outputs at the same time with RPi.GPIO, and pull-ups/pull-downs
- RPi.GPIO cheat sheet
I hope these will be really useful to you. It’s been an intense week putting it all together. Have fun taking over the world, one GPIO port at a time.
RasPiO® GPIO Reference Aids
Our sister site RasPiO has three really useful reference products for Raspberry Pi GPIO work...
Thanks for this Alex – always good to have a cheat sheet at hand!
Would it be possible to include the Fritzing diagrams from parts 4, 5 & 6 into the PDF cheat-sheet too?
Would they make sense without the text?
They would to me ;)
It’s just that I can never remember what resistor values to use in typical circuit setups. But you make a fair point that without the explanatory text they’re possibly confusing.
I had considered making a PDF of the whole series. But right now I need a breather after an intense period of blogging. :)
Take a well-earned break Alex, we wouldn’t want you burning out :)
Heheh, we need to fit a current-limiting resistor to Alex ;-)
LOL – I’m not sure if you do Twitter Andrew? But yesterday I put out a tweet “Would you laugh very hard if I told you I had a series coming up on WiringPi2? for Python”.
I will definitely be taking a much more sedate pace with it though. Some is already written, but there’s plenty more that isn’t.
Nah, I don’t do any social networking sites.
Feel free to drop me a line if you need anything else proof-reading.
Thanks Andrew :)
Thanks for the excellent article on GPIO basics, I am at that point in using the RPi that makes this info timely …… I hope there will be similar tutorials in the future.
Bill RPi web cam, Motion, Apache server, Ext HDD
What is “port_or_pin” means?
Is it pin number?
A very excellent series of articles – thank you. I have a question…
I’ve got Python code below. It works and appropriately lights up the LED’s on my breadboard depending on if the sensors are activated or not.
My starting point wasn’t these articles … it uses gpiozero.
“from gpiozero import LED, Button, MotionSensor”
—-SNIP—-
You are calling “import RPi.GPIO as GPIO”. – This seems to operate at a much lower layer…
The question(s)
… I’m wondering if gpiozero … although it works… is intended for the Raspberry Pi Zero?… and that I should actually be using RPi.GPIO
… and my actual question… is there something I should be reading to understand what these libraries (if that’s what they are called) are intended for?
My Raspberry Pi is a 3B with Ethernet HAT providing POE.
GPIO zero is not intended for Pi Zero, it is intended for all Pi and is meant to be easy to use.
It’s like a layer on top of RPi.GPIO if you like, although I think it no longer depends on RPi.GPIO, it did when it first came out.
It’s simple. GPIO Zero is for beginners and education. It’s easy to use and remember.
RPi.GPIO is slightly ‘lower level’ and gives you a bit more direct control. But it’s not a great deal harder to use for simple port switching.